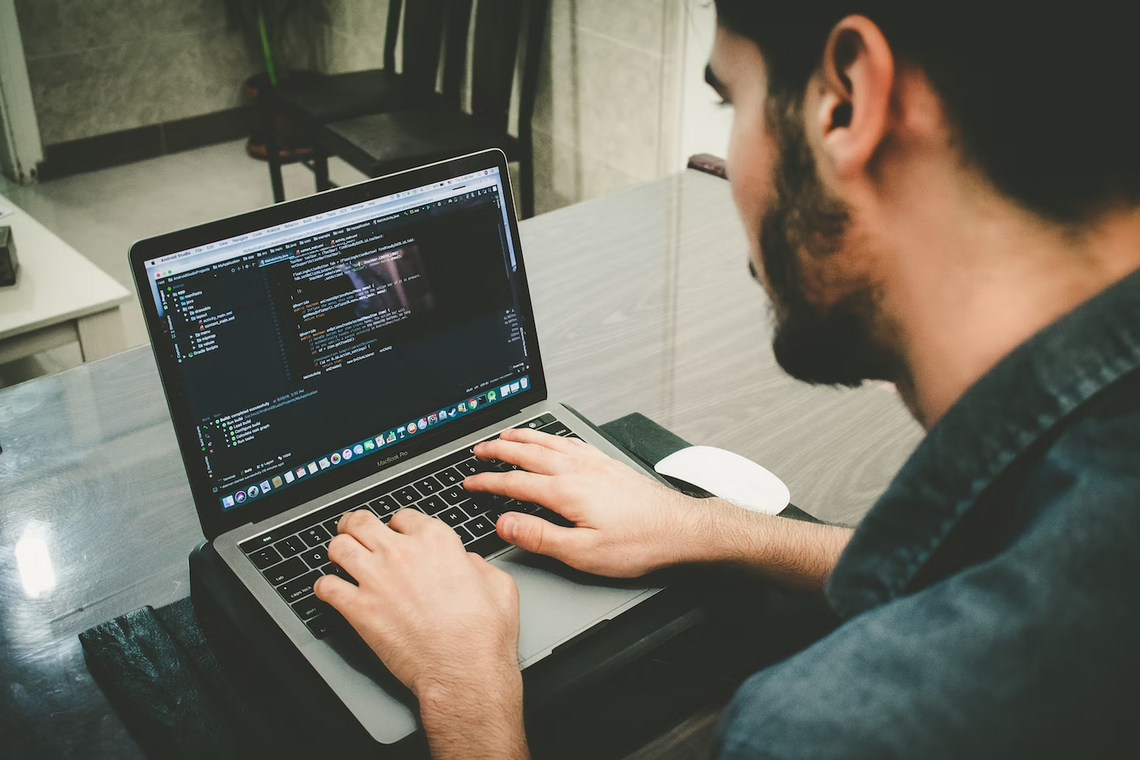
Best practices for handling exceptions in C#
November 18, 2024Error handling is an essential part of software development, especially in languages like C#. Although avoiding errors entirely might seem ideal, it's not always feasible. When errors do occur, how you manage them is crucial to maintaining your program’s functionality and ensuring user satisfaction. Below, we’ll explore some effective practices for error handling in C# that every developer should know.
Understand what error handling is
Before diving into the specifics, it's important to grasp the concept of error handling. It refers to the way a software application reacts and recovers when an error occurs. Essentially, error handling is the process of predicting, detecting, and resolving errors to maintain the normal execution flow of a program.
Errors can range from minor glitches to significant issues that disrupt the application. Effective error handling helps in managing these situations smoothly, preventing crashes and allowing the application to recover when possible.
Always keep track of the exception stack
Maintaining the exception stack is a best practice in error handling. When rethrowing an exception, avoid using throw e; as it erases the original stack trace, making debugging difficult. Instead, use the `throw;` statement within the `catch` block, which preserves the stack trace and provides more accurate information about the error’s origin.
By preserving the stack trace, you'll have a clearer understanding of where the error occurred, which aids in troubleshooting and debugging.
Read on: Mobile app development without coding: Is it really worth considering?
Avoid using "throw ex"
At first glance, it may seem convenient to use `throw ex` after catching an exception, but this approach is problematic. The main issue is that `throw ex` overwrites the original stack trace, preventing developers from tracing the error back to its root cause.
Avoiding it helps to retain the original stack trace and makes debugging easier.
Use multiple catch blocks instead of If conditions
When handling different types of exceptions, it’s better to use multiple `catch` blocks instead of `if` conditions within a single `catch` block. This allows for more precise error handling based on the exception type.
Using specific `catch` blocks for different exceptions improves code readability and ensures that the right actions are taken for each type of error.
Always analyse the caught errors
Catching an error without taking action is a missed opportunity for improvement. Silent exceptions, where errors are caught but ignored, make it impossible to fix the root cause or alert the user. Always analyse and respond to errors, whether by logging them or notifying users.
By taking proactive steps such as logging the error or notifying users, you can improve the user experience and address issues before they escalate.
In conclusion, error handling in C# requires careful consideration and best practices to ensure that your application runs smoothly even when things go wrong. Always preserve the stack trace, avoid overwriting exceptions, use multiple catch blocks, and take action when errors are caught. By following these practices, you’ll create more robust and reliable applications.