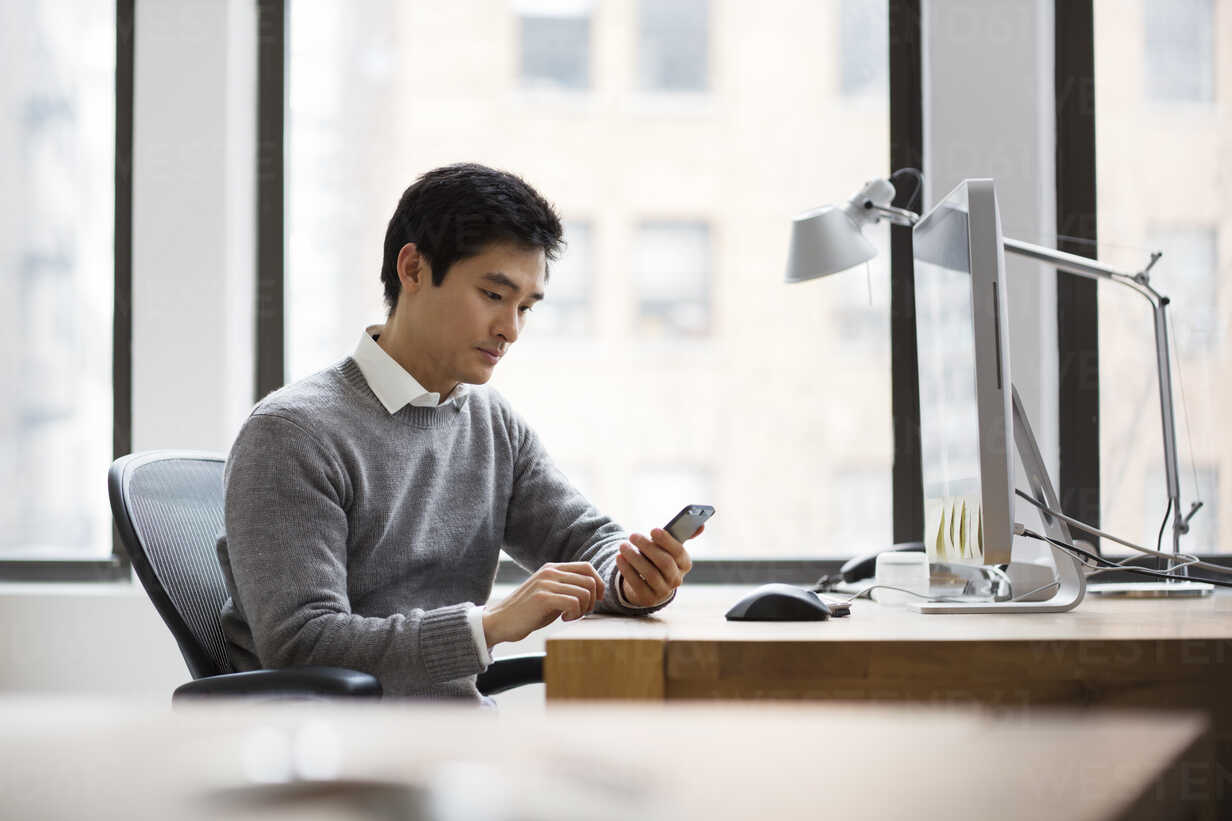
Synchronous vs. asynchronous programming: What’s the difference?
March 17, 2025In software development, two primary programming models—synchronous and asynchronous—govern how code is structured and executed. Understanding these models is essential for choosing the right approach for your projects, as they significantly influence performance, efficiency, and scalability.
Here’s a comprehensive look at the differences, use cases, and decision-making considerations when choosing between synchronous and asynchronous programming.
What is synchronous and asynchronous programming?
Synchronous programming executes tasks sequentially, meaning each task must finish before the next one begins. This model is predictable, straightforward, and ideal for scenarios where tasks depend on the completion of preceding ones.
Asynchronous programming, on the other hand, allows tasks to run independently, enabling multiple processes to occur simultaneously. This approach optimises performance and is particularly useful for managing waiting tasks, such as accessing external servers or APIs.
Learn with us: Understanding the differences between normal classes and data classes in Python.
Key differences
Synchronous programming:
1. Executes tasks in a linear, step-by-step manner.
2. Requires waiting for one task to finish before starting the next.
3. Suitable for applications where precise control and predictability are essential.
4. Less suited for distributed systems or tasks requiring high concurrency.
Asynchronous programming:
1. Enables tasks to run in parallel or independently.
2. Allows code to proceed without waiting for other processes to complete.
3. Ideal for distributed systems and applications requiring multitasking.
4. May introduce complexity in debugging and configuration.
Examples in practice
Imagine writing an application to fetch data from two sources—a continuously updating server and a third-party API.
Synchronous approach:
The application pauses to wait for the server and API responses before continuing, potentially causing delays.
Asynchronous approach:
The application proceeds with other tasks while waiting for the server and API, processing responses as they arrive, and improving efficiency.
Applications in JavaScript
JavaScript, by default, follows a synchronous execution model. However, it offers asynchronous capabilities through features like `setTimeout()`, promises, and async/await functions.
Synchronous use:
Executes scripts sequentially, useful for scenarios needing strict order.
Asynchronous use:
Handles web requests or data loading without blocking the main thread, leveraging event loops for parallelism.
When to choose each model
Asynchronous programming:
1. Best for applications needing to handle multiple simultaneous tasks, such as web development, real-time messaging, or file downloads.
2. Improves scalability and user experience by reducing delays.
Synchronous programming:
1. Ideal for tasks requiring predictable, linear execution, such as game development or data processing.
2. Useful when tasks are interdependent and must be executed in a specific order.
Key Considerations
Complexity:
Asynchronous programming offers efficiency but demands a more intricate setup.
Memory usage:
Synchronous tasks use less memory but may cause delays, while asynchronous tasks require more resources.
Scalability:
Asynchronous programming is better for dynamic, scalable systems with frequent updates.
By understanding the strengths and limitations of each model, developers can make informed decisions tailored to their project's specific requirements. Choosing the right approach ensures optimised performance and a seamless user experience.